Global and Nonlocal Keywords in Python With Best Practices
In Python, the global and nonlocal keywords are used to modify variables in different scopes. These keywords are essential for working with nested functions and accessing/modifying global variables.
It’s important to follow best practices when using them. Specifically, modifying a global variable directly is not recommended. Instead, it’s better to pass the variable as an argument to the function and return the modified value.
In this tutorial, we’ll explore the use of these keywords in detail and discuss best practices for using them.
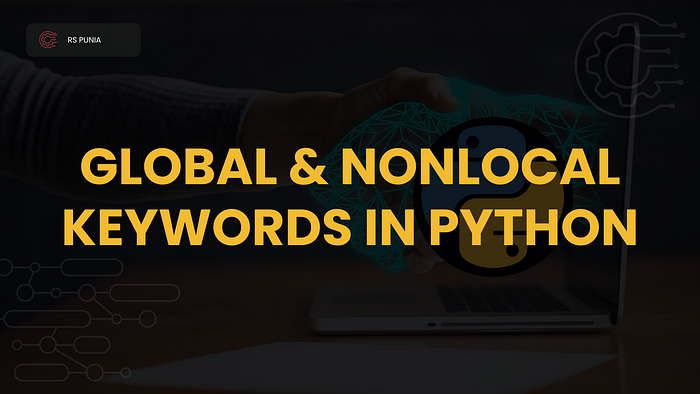
Global Keyword
In Python, the global keyword is used to access and modify global variables within a function. Global variables are variables that can be accessed and modified from any part of the program.
By default, any declared variable is a global variable in Python. We need not explicitly tell Python that we are going to declare a global variable.
For example:
# Declaring global variables
name = 'John'
age = 30
Both name
and age
are global variables, any function declared here will have access to these variables. For example:
name = 'John'
age = 30
def greeting():
print(f"Hello {name}, You are {age} years old!")
greeting()
This will output:
Hello John, You are 30 years old!
Therefore, any function has access to these global variables in Python. But what if we try to modify these variables inside that function?
name = 'John'
age = 30
def greeting():
age = age + 5
print(f"Hello {name}, You are {age} years old!")
greeting()
Here we are trying to modify the variable age by using age = age + 5
. But Python will complain in this case.
It will throw the following error:
UnboundLocalError: local variable 'age' referenced before assignment
To modify a global variable inside a function, we can use the global keyword. Here’s an example:
name = 'John'
age = 30
def greeting():
global age
age = age + 5
print(f"Hello {name}, You are {age} years old!")
greeting()
Output:
Hello John, You are 35 years old!
Global variables should be used sparingly in order to avoid potential issues with unexpected modifications. Instead of directly modifying global variables inside a function, it is generally considered best practice to pass variables as arguments and return the modified value.
This approach can help prevent unintended changes to global variables and promote modularity and reusability in your code. By passing variables as arguments and returning modified values, you can also make your code more flexible and easier to test and debug.
Here’s an example of the recommended approach:
name = 'John'
age = 30
def greeting(age):
age = age + 5
print(f"Hello {name}, You are {age} years old!")
return age
age = greeting(age)
print(f"Updated age: {age}")
Output:
Hello John, You are 35 years old!
Updated age: 35
In this example, we pass the age
variable as an argument to the greeting
function and return the modified value. The original age
variable is then updated with the returned value. This approach avoids modifying the global variable directly inside the function and helps prevent unexpected changes to the global variable.
We use a built-in function globals()
to examine global variables. We will take a closer look at globals()
.
Exploring The Built-in globals() function
The globals()
is a built-in function that returns a dictionary representing the current global symbol table. A global symbol table is a dictionary containing all the global variables that are currently defined in the module. The syntax for the globals() function is as follows:
globals()
This returns a dictionary containing all the global variables currently defined in the module. The following code snippet shows how to use the globals()
function to get all the global variables in the current module.
# global variables
programmer_name = "David"
lang = "Python"
age = 35
print(globals())
Output:
{'__name__': '__main__', '__doc__': None, '__package__': None, '__loader__': <_frozen_importlib_external.SourceFileLoader object at 0x7f6cb196d4c0>, '__spec__': None, '__annotations__': {}, '__builtins__': <module 'builtins' (built-in)>, '__file__': '/home/ram/CodingMantras/medium-blog/test.py', '__cached__': None, 'programmer_name': 'David', 'lang': 'Python', 'age': 35}
In the above example, we have defined three global variables programmer_name
, lang
, and age
. The print(globals())
returns the global symbol table as a dictionary.
Example: Deleting a global variable
The following code snippet shows how to use the globals()
function to delete a global variable.
# global variable
programmer_name= "David"
# function to delete the global variable
def delete_name():
global programmer_name
del globals()["programmer_name"]
# print the current value of the global variable
print(programmer_name)
# delete the global variable
delete_name()
# print the value of the global variable after deletion
print(programmer_name)
Output:
David
Traceback (most recent call last):
File "main.py", line 14, in <module>
print(name)
NameError: name 'name' is not defined
In the above example, we have defined a global variable programmer_name
and a function delete_name()
that deletes the global variable programmer_name
. The global keyword is used inside the function to access the global variable. The del
statement is used to delete the variable from the global symbol table. The print()
function is used to print the current value of the global variable name.
However, it is generally not recommended to delete global variables using functions as it can lead to unexpected behaviour and make the code harder to understand and maintain. Instead, it’s better to use functions to modify the global variable or create new variables within the local scope of the function.
The globals()
function can be useful for debugging and understanding the global variables used in a program.
Nonlocal keyword
The nonlocal keyword is used to access and modify a variable in the outer function from an inner function. It is used to work with nested functions where we need to modify variables defined in an outer function.
Here’s an example:
def outer_function():
num = 10
def inner_function():
nonlocal num
num = 5
print("Inside inner function num:", num)
inner_function()
print("Inside outer function num:", num)
outer_function()
Output:
Inside inner function num: 5
Inside outer function num: 5
In the above example, we have an outer function that defines a variable num
and an inner function that modifies the value of num
using the nonlocal keyword. After calling the inner function, the value of num
is changed both inside and outside the function.
Best practices
- Avoid modifying global variables directly inside a function. Instead, pass the global variable as an argument to the function and then return the modified value.
- Use the nonlocal keyword only when necessary, and avoid using nested functions that modify variables in outer scopes.
- Use meaningful and descriptive variable names to avoid confusion and to make the code more readable.
- Always use local variables instead of global variables whenever possible to avoid name collisions and to make the code more modular and easier to understand.
Conclusion
In this tutorial, we have explored the use of global and nonlocal keywords in Python. We have seen how these keywords can be used to modify variables in different scopes, along with best practices to use them safely. By following these best practices, we can write more readable and maintainable code that is less prone to errors and unexpected behaviour.
Hey there👋! If you found this tutorial helpful, feel free to show your appreciation by clapping for it! Remember, you can clap multiple times if you really like it.
